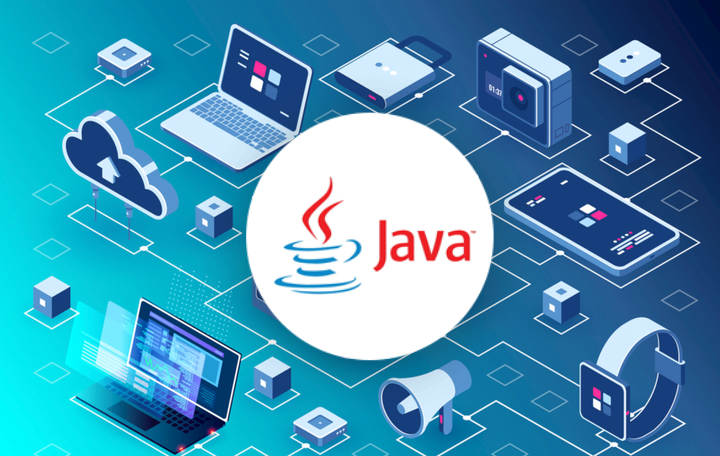
Securing Your Java Backend Applications: Best Practices for Authentication and Authorization
Introduction
In the realm of Java development, securing applications is a paramount concern that developers face. Authentication and authorization mechanisms are the bedrock of application security, ensuring that only legitimate users can access the system and perform actions within their granted permissions. This blog post delves into the best practices for implementing robust authentication and authorization in Java applications.
Security in backend applications is a fundamental pillar for data protection and user trust. In today’s digital world, where cyber threats are constantly evolving, securing backend applications has become an imperative need. Authentication and authorization are two critical aspects of security that help verify the identity of users and ensure that they only have access to the resources they are allowed to.
The lack of robust security measures in authentication and authorization can lead to a variety of risks, including unauthorized access to sensitive information, data alteration, and man-in-the-middle attacks, where an attacker can intercept communication between the user and the system. In addition, security vulnerabilities can damage a company’s reputation, result in significant financial losses, and expose users to fraud and identity theft.
Securing backend applications in Java is a complex but essential task that requires a combination of advanced technologies, best practices, and a security-conscious culture. By adopting a proactive and security-centric approach, organizations can effectively protect their systems and data from emerging cyber threats.
Understanding Authentication and Authorization
Authentication is the process of verifying the identity of a user or entity. In Java, this can be achieved through various means such as username/password, tokens, or biometric data. Authorization, on the other hand, determines what an authenticated user is allowed to do. It involves assigning roles and permissions that control access to resources within the application.
Java Authentication and Authorization Service (JAAS)
Java Authentication and Authorization Service (JAAS) is a powerful technology that enables Java applications to not only authenticate, meaning verify the identity of users, but also authorize, determining whether a user has access to certain resources or operations. JAAS is based on a pluggable security model, which means it can be adapted to use different authentication providers without requiring substantial changes to the application code. This makes it extremely versatile and useful in enterprise environments where security requirements can be complex and ever-changing.
JAAS operates primarily in two phases: authentication and authorization. During authentication, JAAS verifies the user’s credentials, such as usernames and passwords, against a security store, which can be a database, an LDAP directory, or any other credential storage system. Once the user’s identity has been confirmed, JAAS proceeds to the authorization phase. At this stage, JAAS uses security policies to determine whether the user has the necessary permissions to perform the requested action in the application.
One of the key features of JAAS is its ability to be customized and extended. Developers can create their own authentication and authorization modules to meet specific needs. In addition, JAAS integrates well with other Java security technologies, such as Java Secure Socket Extension (JSSE) and Java Cryptography Extension (JCE), providing a comprehensive security solution for Java applications.
Here’s a more detailed explanation of the authentication and authorization phases in JAAS:
Authentication:
-
Login: The user enters their credentials (e.g., username and password) into the application.
-
Callback Handler: The application invokes a callback handler, which prompts the user for their credentials if they haven’t already been provided.
-
Login Module: The callback handler passes the credentials to a login module, which is responsible for verifying the user’s identity.
-
Authentication Success: If the credentials are valid, the login module returns a successful authentication result.
-
Authentication Failure: If the credentials are invalid, the login module returns an authentication failure result.
Authorization:
-
Subject: The authenticated user is represented by a Subject object.
-
Access Control List (ACL): The application checks the Subject’s permissions against an access control list (ACL) associated with the resource or operation.
-
Permission Granted: If the Subject has the necessary permissions, access is granted.
-
Permission Denied: If the Subject lacks the required permissions, access is denied.
JAAS provides a robust and flexible framework for implementing authentication and authorization in Java applications. Its pluggable architecture, customizable modules, and integration with other Java security technologies make it a valuable tool for securing enterprise Java applications.
Spring Security
Spring Security is a powerful and highly customizable authentication and authorization framework for Java applications. It provides comprehensive security features that are easy to implement in Spring-based applications. Spring Security handles everything from login/logout functionality to protection against common exploits like Cross-Site Request Forgery (CSRF).
Best Practices for Authentication
Token-based Authentication
Token-based authentication is a crucial strategy for safeguarding Java applications. Tokens serve as digital credentials for users, representing small pieces of information. They function by assigning a unique token to each user session, which is then used to verify the user’s identity for every request made to the server. This technique offers several advantages, including reducing the need to store passwords on the server, thereby mitigating the risk of brute-force attacks and credential theft.
Implementing token-based authentication in Java can be achieved using various libraries and frameworks, such as Spring Security. Spring Security provides a robust set of tools for efficiently managing security. A code example might involve creating an authentication filter that intercepts requests and verifies the token’s presence and validity. This process typically entails decoding the token, validating its signature, and ensuring the token hasn’t expired.
Furthermore, it’s essential to ensure secure token generation, employing strong encryption algorithms and storing them securely on the client-side, such as in an HTTP cookie inaccessible to client-side scripts (HttpOnly cookie). Additionally, implementing a token renewal mechanism is crucial to maintain security in case a token is compromised.
Token-based authentication is a recommended practice for Java application security as it provides an extra layer of protection and more efficient user session management. With careful implementation and the use of appropriate tools, a robust and secure authentication system can be achieved.
A practical example of token-based authentication in Java:
Scenario:
Consider a web application that allows users to manage their finances. To protect user accounts and transactions, the application implements token-based authentication.
Implementation:
-
User Login:
- Upon successful login, the server generates a unique JSON Web Token (JWT) for the user.
- The JWT contains essential information like user ID, expiration time, and other claims.
- The JWT is sent back to the client (web browser) in the response.
-
Client-side Storage:
- The client securely stores the JWT in a dedicated cookie (HttpOnly cookie) to prevent JavaScript access.
-
Subsequent Requests:
- For each subsequent request to the server, the client includes the JWT in the authorization header.
-
Server-side Validation:
- The server extracts the JWT from the authorization header.
- The server verifies the JWT’s signature to ensure its authenticity and integrity.
- The server validates the JWT’s claims, including expiration time and user identity.
- If the JWT is valid, the server grants access to the requested resource.
- If the JWT is invalid or expired, the server returns an unauthorized error.
-
Token Refresh:
- To maintain session validity, the client can periodically refresh the JWT before it expires.
- The client sends a refresh request to the server, including the current JWT.
- If the current JWT is still valid, the server generates a new JWT and sends it back to the client.
- The client updates the stored JWT with the new one.
Benefits:
- Reduced Password Storage: Tokens eliminate the need to store passwords on the server, reducing the risk of data breaches.
- Improved Security: Tokens provide a secure mechanism for verifying user identity without exposing passwords.
- Stateless Authentication: Tokens are self-contained, enabling stateless authentication and scalability.
- Session Management: Tokens facilitate effective session management and expiration control.
Example Code (Spring Security):
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtTokenProvider jwtTokenProvider;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/login").permitAll()
.anyRequest().authenticated()
.and()
.apply(jwtConfigurer());
}
private JwtConfigurer jwtConfigurer() {
return new JwtConfigurer(jwtTokenProvider);
}
}
public class JwtTokenProvider {
private final String secretKey;
public JwtTokenProvider(String secretKey) {
this.secretKey = secretKey;
}
public String generateToken(UserDetails userDetails) {
// Create a JWT token with user claims
// Set expiration time
// Sign the token using the secret key
return token;
}
public boolean validateToken(String token) {
// Parse the token
// Extract claims
// Verify signature using the secret key
// Check if token is expired
return valid;
}
// Additional methods for retrieving user details from token and refreshing tokens
}
Delegated Authentication
Implementing secure authentication protocols like OAuth 2.0 and OpenID Connect in Java involves several critical steps to ensure the application handles authentication and authorization effectively and securely. First, it is essential to understand the fundamentals of these protocols. OAuth 2.0 is an industry standard for authorization that allows applications to gain limited access to user accounts on an HTTP server, while OpenID Connect is an identity layer built on top of OAuth 2.0 that enables applications to verify the end-user’s identity based on the authentication performed by an authorization server.
To begin, a Java library compatible with these protocols must be selected, such as Keycloak, an open-source authentication server that offers comprehensive support for OAuth 2.0 and OpenID Connect. It is important to review the documentation of the chosen library to understand how to integrate it into the existing Java application. Configuring Keycloak, for instance, involves defining clients and roles, as well as setting up authentication and authorization flows according to the application’s specific needs.
The next step is to configure the authorization server to handle authentication and authorization requests. This includes establishing security policies, configuring secure redirect domains, and registering client applications that will be authorized to access the server’s resources. Additionally, it is crucial to implement proper token management, including the issuance, renewal, and revocation of access tokens and refresh tokens.
On the client-side, the Java application must be able to redirect users to the authorization server for login and then handle the server’s response, which will include an access token upon successful authentication. This access token will be used to make requests to protected resources, ensuring that only authenticated and authorized users can access them.
To ensure security, it is essential to implement protective measures against common attacks, such as Cross-Site Request Forgery (CSRF) attacks and session hijacking. It is also recommended to use HTTPS for all communication between the client and the server to protect the confidentiality and integrity of transmitted data.
Finally, it is important to conduct thorough testing to ensure the implementation is secure and functions as expected. This includes penetration testing to identify and mitigate potential security vulnerabilities.
A practical examples:
Scenario:
Consider a web application that manages user accounts and provides access to protected resources. To enhance security and improve user experience, the application will implement OAuth 2.0 and OpenID Connect for authentication and authorization.
Implementation Steps:
-
Choose a Java library: Select a Java library that supports OAuth 2.0 and OpenID Connect. A popular option is Keycloak, an open-source authentication server that offers comprehensive support for these protocols.
-
Set up Keycloak: Install and configure Keycloak to serve as the identity provider (IdP). This involves defining clients, roles, and configuring authentication and authorization flows based on the application’s requirements.
-
Configure the Client Application: Integrate the Keycloak library into the Java web application. This involves creating a Keycloak client instance, configuring client details, and establishing communication with the Keycloak server.
-
Implement User Authentication: Implement a login mechanism in the web application that redirects users to the Keycloak server for authentication. Upon successful authentication, Keycloak redirects the user back to the application with an authorization code.
-
Exchange Authorization Code for Access Token: The web application exchanges the authorization code received from Keycloak for an access token. This involves sending a POST request to the Keycloak token endpoint, including the authorization code and client credentials.
-
Secure Resource Access: Use the access token obtained from Keycloak to make authorized requests to protected resources. The access token is typically included in the Authorization header of the HTTP request.
-
Handle User Profile Information: Retrieve user profile information from Keycloak using the access token. This involves sending a GET request to the Keycloak userinfo endpoint, including the access token.
-
Implement Security Measures: Implement security measures to protect against common attacks, such as CSRF attacks and session hijacking. Use HTTPS for all communication between the client and the server.
-
Thorough Testing: Conduct thorough testing to ensure the implementation is secure and functions as expected. This includes penetration testing to identify and mitigate potential security vulnerabilities.
Example Code Snippets:
Keycloak Client Configuration:
// Keycloak client configuration
KeycloakSecurityContext context = Keycloak.getContext();
AccessToken accessToken = context.getToken();
String userId = accessToken.getSubject();
String userName = accessToken.getName();
String email = accessToken.getEmail();
Accessing Protected Resources:
// Accessing protected resources
String accessToken = "Bearer " + context.getToken().getStringValue();
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.add("Authorization", accessToken);
ResponseEntity<String> response = restTemplate.exchange(
"https://protected-resource.com/api/data",
HttpMethod.GET,
null,
String.class,
headers
);
Best Practices for Authorization
In the field of software development, particularly in enterprise applications, authorization and security are crucial aspects that must be handled with care. Role-Based Access Control (RBAC) is an authorization approach that simplifies permission management by assigning roles to users and then linking those roles to specific permissions. This is particularly important in large applications where permissions need to be clearly defined and centrally managed.
Implementing RBAC in Java can be achieved through various frameworks and libraries, such as Spring Security, Apache Shiro, or even EJB containers. These frameworks offer an abstraction over implementation details and allow developers to focus on business logic, ensuring that security principles remain consistent throughout the application.
For effective permission management, it is crucial to establish clear security policies and maintain detailed documentation of roles and permissions. This not only facilitates auditing and compliance with regulations but also helps prevent excessive permission assignment, a common problem known as “privilege creep.”
Furthermore, it is recommended to use tools and libraries that are widely adopted and maintained by the community, as this ensures ongoing support and updates against emerging security vulnerabilities. Tools like JAAS (Java Authentication and Authorization Service) provide a robust framework for authentication and authorization in Java applications.
Example code:
// RBAC example
import org.springframework.security.access.annotation.Secured;
import org.springframework.stereotype.Service;
@Service
public class ProjectService {
// Only users with the 'ADMIN' role can execute this method
@Secured("ROLE_ADMIN")
public void createProject(Project project) {
// Logic to create a new project
}
// Users with 'ADMIN' or 'USER' roles can execute this method
@Secured({"ROLE_ADMIN", "ROLE_USER"})
public Project getProjectById(Long projectId) {
// Logic to get a project by its ID
return new Project();
}
}
- Use of Annotations: Use annotations like @Secured to define the roles that can access specific methods.
- Security Frameworks: Implement authorization with frameworks like Spring Security that offer support for RBAC.
- Documentation and Policies: Maintain clear documentation of roles and permissions to facilitate auditing and prevent “privilege creep”.
- Community Tools: Choose libraries like JAAS that have strong community support and regular updates against vulnerabilities.
Encryption: Protecting Data at Rest and in Transit
Encryption is an essential tool for protecting data both at rest and in transit, ensuring that sensitive information remains inaccessible to unauthorized actors. The importance of encryption lies in its ability to convert readable data into an encoded format that can only be deciphered by those who possess the corresponding key, which is crucial for maintaining data confidentiality and integrity in a digital world where security threats are omnipresent and constantly evolving.
There are several common encryption methods used to protect information. Symmetric algorithms like AES and DES use the same key to encrypt and decrypt data, making them fast and efficient for processing large volumes of information. On the other hand, asymmetric algorithms, such as RSA, employ a pair of keys, a public key and a private key, facilitating secure key distribution and essential for secure communication over the internet and other networks.
Aside from the commonly mentioned symmetric and asymmetric encryption algorithms, there are various other encryption methods applicable to Java backends. Here’s a brief overview of some notable options:
-
Hashing: Hashing functions, such as SHA-256 and MD5, are used to generate a unique fingerprint or digest of data. They are not reversible, meaning the original data cannot be retrieved from the hash value. Hashing is often employed for data integrity verification, password storage, and digital signatures.
-
Digital Signatures: Digital signatures provide a mechanism to verify the authenticity and integrity of a message or document. They involve using a private key to generate a signature that is then attached to the message. The recipient can verify the signature using the corresponding public key, ensuring that the message has not been tampered with and originated from the claimed sender.
-
Key Wrapping: Key wrapping techniques, like using RSA, are used to encrypt cryptographic keys for secure storage or transmission. The encrypted key can only be decrypted by the intended recipient using their private key. This ensures that sensitive keys are protected even if the storage or transmission medium is compromised.
-
Homomorphic Encryption: Homomorphic encryption allows for computations to be performed on encrypted data without revealing the plaintext. This enables secure data sharing and processing while maintaining privacy. However, homomorphic encryption is computationally intensive and may not be suitable for all scenarios.
-
Attribute-Based Encryption (ABE): ABE provides fine-grained access control by encrypting data with attributes and allowing decryption only to users possessing the corresponding attributes. This enables flexible access control policies and can be useful for scenarios with multiple users and varying access requirements.
-
Secure Multi-party Computation (SMPC): SMPC enables multiple parties to collaboratively compute a function on their private data without revealing their individual inputs. This allows for secure data analysis and collaboration without compromising privacy. SMPC is particularly relevant in fields like finance and healthcare where sensitive data needs to be processed jointly.
-
Fully Homomorphic Encryption (FHE): FHE is a more advanced form of homomorphic encryption that supports arbitrary computations on encrypted data. It offers the strongest level of privacy but is also computationally demanding. FHE is still an area of active research, but it holds potential for secure cloud computing and data analytics.
The choice of encryption method depends on the specific requirements of the application, considering factors like security level, performance, and flexibility. A combination of techniques may be employed to achieve a comprehensive security solution.
Best practices for encryption key management include regular key rotation, the use of secure key stores, and the implementation of restricted access policies to minimize the risk of compromise. It is vital that keys are stored and handled securely to maintain the integrity of encrypted data. Additionally, adopting security protocols like TLS and SSL for data encryption in transit helps protect against interception and unauthorized access during data transmission.
Encryption is an indispensable security measure that plays a crucial role in protecting data at rest and in transit. Its proper implementation and effective key management are fundamental to ensuring data security in today’s cybersecurity landscape. With the growing reliance on technology and the digitalization of information, encryption will continue to be a vital component in the security strategy of any organization.
Aditional Tips
- Use Strong Authentication Mechanisms: Implement multi-factor authentication and use strong, hashed passwords.
- Principle of Least Privilege: Assign the minimal level of access necessary for users to perform their tasks.
- Regularly Update Security Dependencies: Keep all security-related dependencies up-to-date to protect against known vulnerabilities.
- Input Validation: Validate all user input to prevent common vulnerabilities such as SQL injection and cross-site scripting (XSS).
- Use HTTPS: Encrypt data in transit using HTTPS to prevent man-in-the-middle attacks.
- Session Management: Implement secure session management practices, including secure cookie attributes and session expiration.
- Implement OAuth2 for Stateful and Stateless Authentication: OAuth2 is a protocol that allows applications to secure designated access without having to handle user credentials directly, providing a more secure authentication process.
Conclusion
In the world of Java application development, the implementation of authentication and authorization mechanisms stands as a paramount concern. It is a foundational aspect of security that safeguards user data and governs access to resources. Utilizing established frameworks such as JAAS (Java Authentication and Authorization Service) and Spring Security enables developers to construct robust security infrastructures. These frameworks offer a plethora of features like user authentication, authorization, session management, and password encryption, which are essential for creating a secure environment.
Authentication, the process of verifying the identity of a user or entity, is the first line of defense against unauthorized access. It ensures that users are who they claim to be. Authorization, on the other hand, determines whether a user has the right to access certain resources or perform specific actions within the application. It is about granting the appropriate level of access to authenticated users, thus preventing privilege escalation and data breaches.
Best practices in security dictate that developers should not only implement these systems but also continuously monitor and update them to counteract emerging threats. Security is an ongoing endeavor that must adapt to the ever-changing landscape of cyber threats. Regular updates, patches, and security audits are crucial in maintaining the integrity of an application’s security posture.
Moreover, the community and online resources serve as invaluable assets for developers seeking to enhance their understanding of security implementations. The wealth of tutorials, documentation, and community forums provide a rich source of knowledge and examples that can guide developers through the intricacies of Java security.
To resume, the integration of authentication and authorization in Java applications is not merely a feature but a necessity. It is a commitment to the protection of user data and the overall security of the application. As developers, it is our responsibility to stay informed, utilize the best tools available, and remain vigilant in our security practices. The journey towards a secure application is continuous, and with the right approach and resources, developers can fortify their applications against the multitude of cyber threats. Let us code with security in mind, always striving to protect and serve the users who entrust us with their data. Stay secure and code responsibly.